Mastering npm: A Comprehensive Guide for Developers
npm is a key part of the Node.js ecosystem and is widely used in the development of modern web applications, especially those using JavaScript frameworks like React, Angular, and Vue.js.
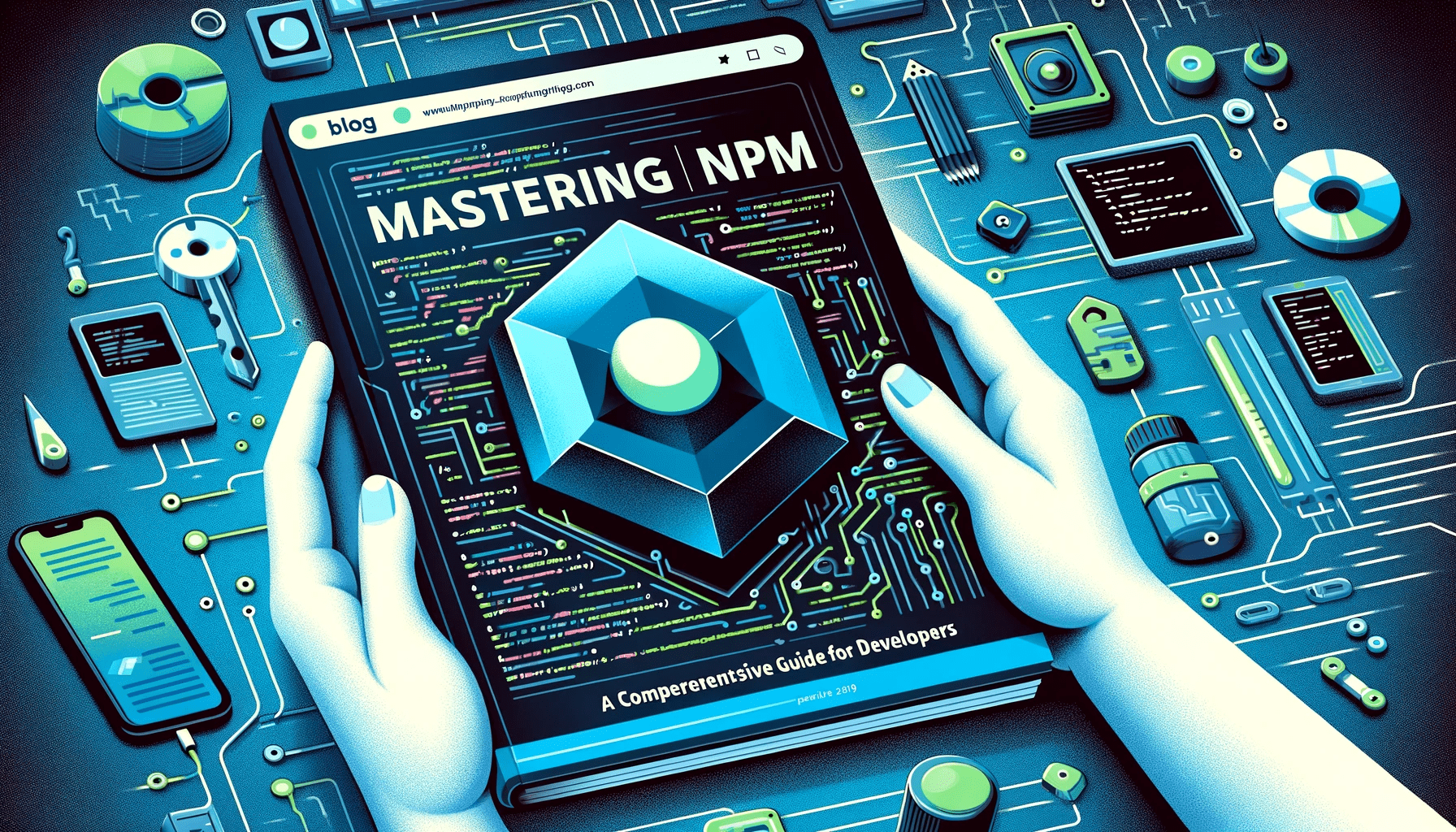
In the dynamic world of web development, npm stands as an indispensable tool for developers. It streamlines project dependencies and package management, significantly enhancing development efficiency. This guide dives into the essentials of npm, providing a clear roadmap from installation to advanced package management.
Table of Contents
- What is npm?
- Install Node.js
- Create a Project Folder
- Launch npm
- Install npm Packages
- Understanding Terminal Command Keys
- Observing npm Events
- Conclusion
What is npm?
NPM stands for Node Package Manager, and it is the default package manager for the JavaScript runtime environment Node.js. It consists of a command line client (also called npm), and an online database of public and private packages, called the npm registry. The registry is accessed via the client, and the available packages can be browsed and searched via the npm website.
npm is a key part of the Node.js ecosystem and is widely used in the development of modern web applications, especially those using JavaScript frameworks like React, Angular, and Vue.js.
Step 1: Install Node.js
First and foremost, install Node.js from its official website. npm, a package manager, comes bundled with Node.js, making its installation mandatory. To check if you already have Node.js, and to ensure it's the latest version, use:
node -v
npm -v
Terminal
Step 2: Create a Project Folder
Organizing your work into dedicated project folders is crucial. It helps manage multiple projects efficiently. Create a folder using the terminal:
mkdir your-project-name
Terminal
To confirm creation, use ls
. Alternatively, you can create a folder using your system's graphical interface.
Step 3: Launch npm
For new projects, begin by generating a package.json
file:
npm init
Terminal
This file is pivotal, outlining your project's dependencies and metadata.
Step 4: Install npm Packages
Now, let's install a package. For instance, ESLint, a popular linting tool:
npm i eslint --save-dev --save-exact
Terminal
But, what do these additional commands mean?
Step 5: Understanding Terminal Command Keys
--save-dev
adds the package under devDependencies
in package.json
, signifying its use in development only. --save-exact
ensures the exact version is installed, fostering consistency across development environments. Shortcuts like -D
(for --save-dev
) and -E
(for --save-exact
) exist:
npm i eslint -DE
Terminal
Step 6: Observing npm Events
Upon installation, observe these changes:
package-lock.json
appears, logging exact versions of installed packages.devDependencies
inpackage.json
updates with the new package.- A
node_modules
folder is created, housing all installed packages.
Remember, commit package-lock.json
but exclude node_modules
by adding it to .gitignore
.
Scripting in npm
Utilize the scripts
section in package.json
for custom commands. For example:
{
"scripts": {
"lint": "eslint"
}
}
Terminal
Running npm run lint
activates ESLint, a tool that helps identify and fix issues in your JavaScript code.
Conclusion
This guide demystifies npm, from initial setup to understanding complex commands. By embracing these practices, you'll enhance your project's structure and efficiency.
Stay tuned for more insights into npm's advanced features and happy coding!