What is Section Rendering API in Shopify?
Shopify Section Rendering API allows you to dynamically update the content of sections on your store's pages without fully reloading the page.
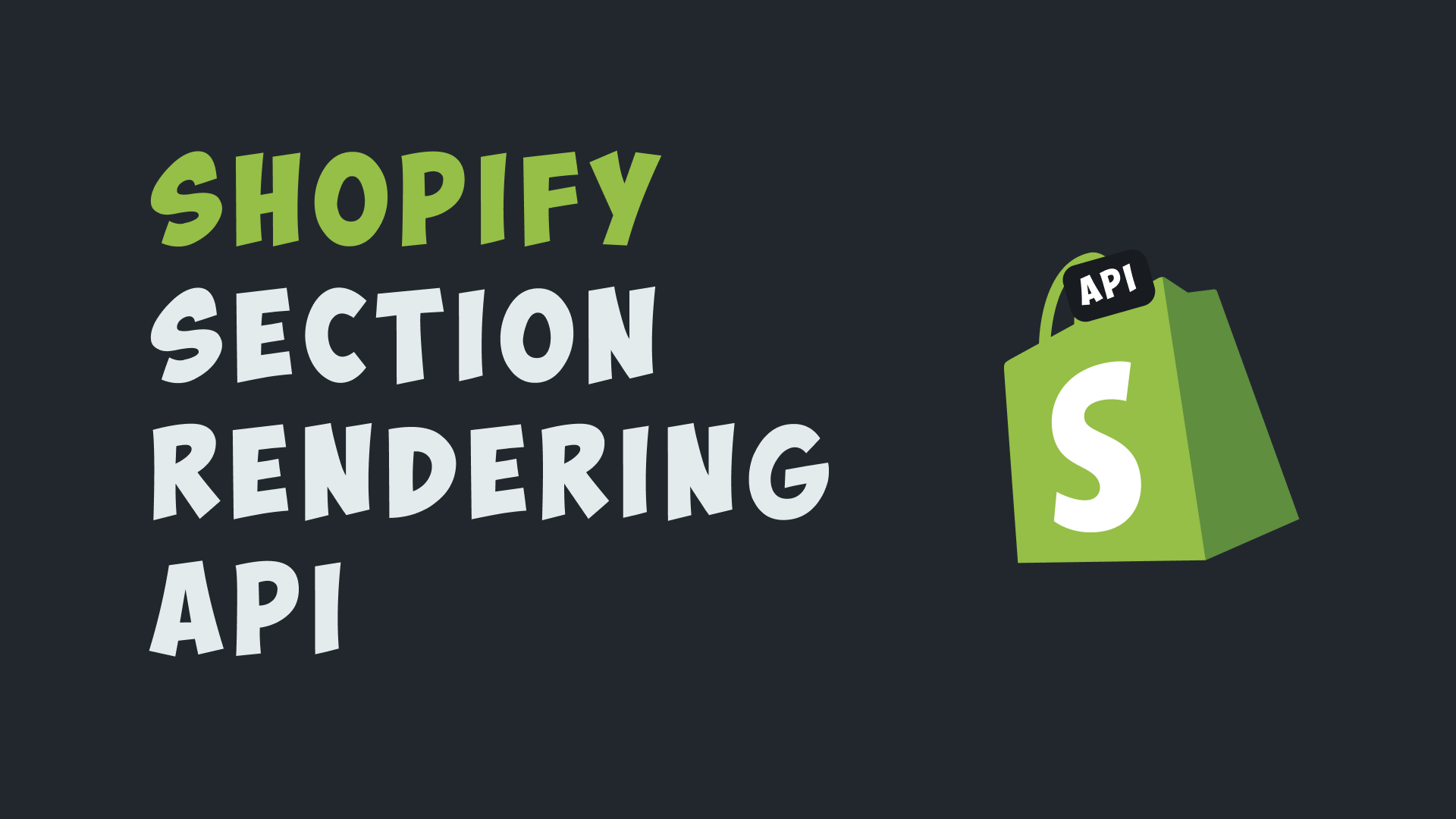
The Section Rendering API in Shopify is an API that allows you to dynamically update the content of sections on your store's pages without fully reloading the page. This is especially useful for creating interactive elements like filters, cart updates, product variant switching, and more.
Key features of the Section Rendering API:
- Asynchronous Updates: You can send a request to the server to retrieve updated content for a specific section. The server returns HTML, which can then be embedded into the current page without a full reload.
- Performance Improvement: Since only a specific part of the page is updated, this can significantly enhance performance and the user experience.
- Flexibility and Control: You can use this API to update individual sections of a page, giving you more control over how and when data on the page is updated.
- Ease of Use: API requests are made via AJAX, and the resulting HTML is easily integrated into the DOM using JavaScript.
A practical use case for the Section Rendering API includes:
- updating the cart contents after adding an item
- filtering products on a collection page,
- changing a product variant on a product page where only specific sections are updated to reflect the new information.
This API helps create a more dynamic and responsive interface for users of Shopify stores.
You can see the example of using Shopify Section Rendering API in action below.
HTML
<div id="cart-section">
{% section 'cart-template' %}
</div>
<button id="add-to-cart" data-product-id="{{ product.id }}">
Add to Cart
</button>
JS
document.getElementById('add-to-cart').addEventListener('click', function() {
const productId = this.getAttribute('data-product-id');
// Add the product to the cart via AJAX
fetch('/cart/add.js', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
id: productId,
quantity: 1,
}),
})
.then(response => response.json())
.then(() => {
// Fetch the updated cart section
fetch('/?sections=cart-template')
.then(response => response.json())
.then(data => {
// Update the cart section with the new HTML
document.getElementById('cart-section').innerHTML = data['cart-template'];
});
})
.catch(error => {
console.error('Error adding to cart:', error);
});
});